本文最后更新于:2023年3月19日 晚上
Lt117. 填充每个节点的下一个右侧节点指针 II
给定一个二叉树
1 2 3 4 5 6
| struct Node { int val; Node *left; Node *right; Node *next; }
|
填充它的每个 next 指针,让这个指针指向其下一个右侧节点。如果找不到下一个右侧节点,则将 next 指针设置为 NULL
。
初始状态下,所有 next 指针都被设置为 NULL
。
进阶:
- 你只能使用常量级额外空间。
- 使用递归解题也符合要求,本题中递归程序占用的栈空间不算做额外的空间复杂度。
示例:
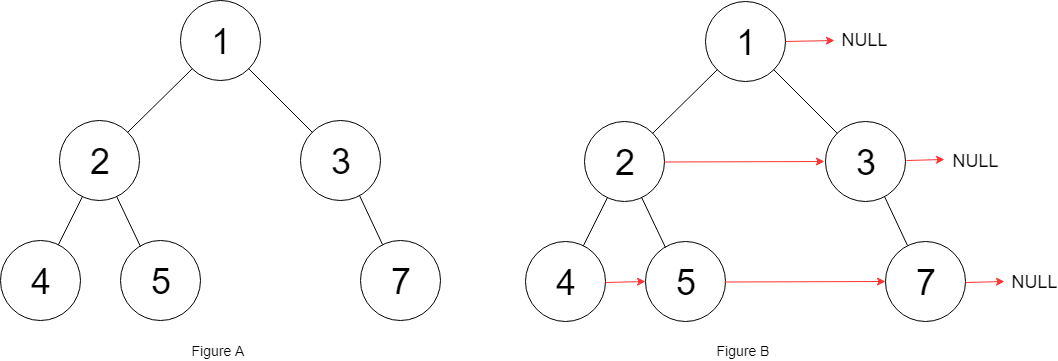
1 2 3
| 输入:root = [1,2,3,4,5,null,7] 输出:[1,#,2,3,#,4,5,7,#] 解释:给定二叉树如图 A 所示,你的函数应该填充它的每个 next 指针,以指向其下一个右侧节点,如图 B 所示。
|
提示:
- 树中的节点数小于
6000
-100 <= node.val <= 100
思路
队列 BFS
常规 BFS,空间复杂度最大应该为满二叉树,最后一层占用队列长度应该为,
,n 为节点数。
和普通层次遍历不同的是,在遍历当前层节点时,将当前节点的 next 指向下一个节点。
解答
队列 BFS
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
|
var connect = function (root) { if (!root) return null; const temp = []; temp.push(root); while (temp.length) { const temp2 = temp.splice(0, temp.length); while (temp2.length) { const node = temp2.shift(); node.next = temp2.length > 0 ? temp2[0] : null; if (node && node.left) temp.push(node.left); if (node && node.right) temp.push(node.right); } } return root; };
|