本文最后更新于:2023年3月19日 晚上
Lt1184. 公交站间的距离
环形公交路线上有 n
个站,按次序从 0
到 n - 1
进行编号。我们已知每一对相邻公交站之间的距离,distance[i]
表示编号为 i
的车站和编号为 (i + 1) % n
的车站之间的距离。
环线上的公交车都可以按顺时针和逆时针的方向行驶。
返回乘客从出发点 start
到目的地 destination
之间的最短距离。
示例 1:
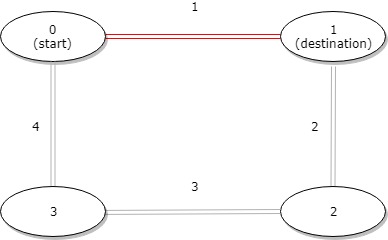
1 2 3
| 输入:distance = [1,2,3,4], start = 0, destination = 1 输出:1 解释:公交站 0 和 1 之间的距离是 1 或 9,最小值是 1。
|
示例 2:
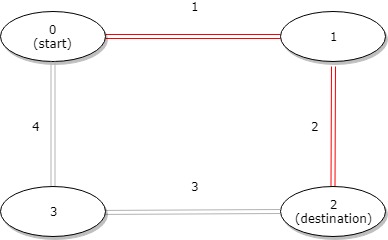
1 2 3
| 输入:distance = [1,2,3,4], start = 0, destination = 2 输出:3 解释:公交站 0 和 2 之间的距离是 3 或 7,最小值是 3。
|
示例 3:
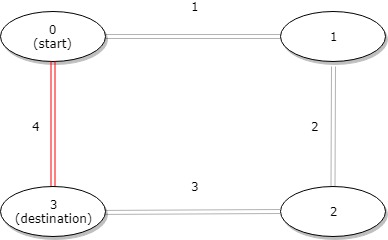
1 2 3
| 输入:distance = [1,2,3,4], start = 0, destination = 3 输出:4 解释:公交站 0 和 3 之间的距离是 6 或 4,最小值是 4。
|
提示:
1 <= n <= 10^4
distance.length == n
0 <= start, destination < n
0 <= distance[i] <= 10^4
思路
先保证 start 一定小于 destination,遍历数组,其实题目就转换成了求一个数组分成三段后,再比较中间一段的长度和两边两段长度之和,取较短的那一方即可。
解答
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
|
var distanceBetweenBusStops = function (distance, start, destination) { let distance1 = 0, distance2 = 0; if (start > destination) [start, destination] = [destination, start]; for (let i = 0; i < distance.length; i++) { if (i >= start && i < destination) { distance1 += distance[i]; } else { distance2 += distance[i]; } } return Math.min(distance1, distance2); };
|